Hibernate and Spring Data JPA: Beginner to Guru
Loại khoá học: Web Development
Master Hibernate, Remove the mystery of Spring Data JPA - Use Spring Boot 3!
Mô tả
Hibernate is the default JPA implementation used by Spring Data JPA.
NOTE: Java 17 and Spring Boot 3 are required for this course.
JPA stands for Java Persistence API. This is a common Java API used to work with Relational Databases.
Spring Data JPA is an abstraction built on top of the JPA API specification.
Being an abstraction, Spring Data JPA makes working with database entities very efficient.
Spring Data JPA eliminates a lot of the boilerplate / cerimonial code, and allows developers to focus on developing business logic.
The downside of the efficient abstraction is that accessing the database can become a mystery. Developers who just understand how to use Spring Data JPA do not understand the complexities of JDBC and Hibernate.
You will start this course with a basic demonstration of Spring Data JPA. In this section you will learn how to work with a H2 in-memory database.
You'll see how easy it is to work with Spring Data JPA. You will also begin to understand how the Hibernate interaction is being abstracted away.
Since JPA is the Java API for working with Relational Databases, the course takes a closer look at Relational Databases and MySQL specifically.
MySQL is the most popular open source relational database in the world. You will learn how to configure Spring Boot to test with a H2 in-memory database and to run integration tests against a MySQL database. This is a common real-world example leveraging the power of Spring and Hibernate to give you a very flexible environment.
Once we've established a persistent database, we can explore using database migration tools.
Liquibase and Flyway are two very popular database migration tools. Spring Boot supports both options. And you will learn about both options and database security best practices.
By establishing a MySQL database, Spring Boot Integration Tests, and automated database migrations we can use Test Driven Development to explore the features of JDBC and Hibernate.
In the course you will learn:
What is the DAO pattern, and how to implement it using JDBC, Spring's JDBCTemplate, and Hibernate
Relational Database Principles
Schema Creation in MySQL
Schema Generation using Hibernate
Database Migrations using Liquibase
Database Migrations using Flyway
Database Integration Testing using Spring Boot and JUnit 5
Defining Primary Key's with Hibernate
Hibernate Criteria Queries
Named JPA Queries
Spring Data JPA query methods
Spring Data JPA @Query Annotation
Entity Relationships - One to One, One to Many, Many to One, Many to Many
Embedded Types
Natural Keys
Composite Keys
Spring Data JPA Query Methods
Paging and Sorting
Database Transaction Management
Database Fetch Operations
Data Validation
JPA Inheritance
Hibernate Interceptors and Listeners
JPA Callbacks
Legacy Database Mapping
Using Multiple Data Sources
Spring Data REST
Learn Hibernate and Spring Data JPA - Enroll today!
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
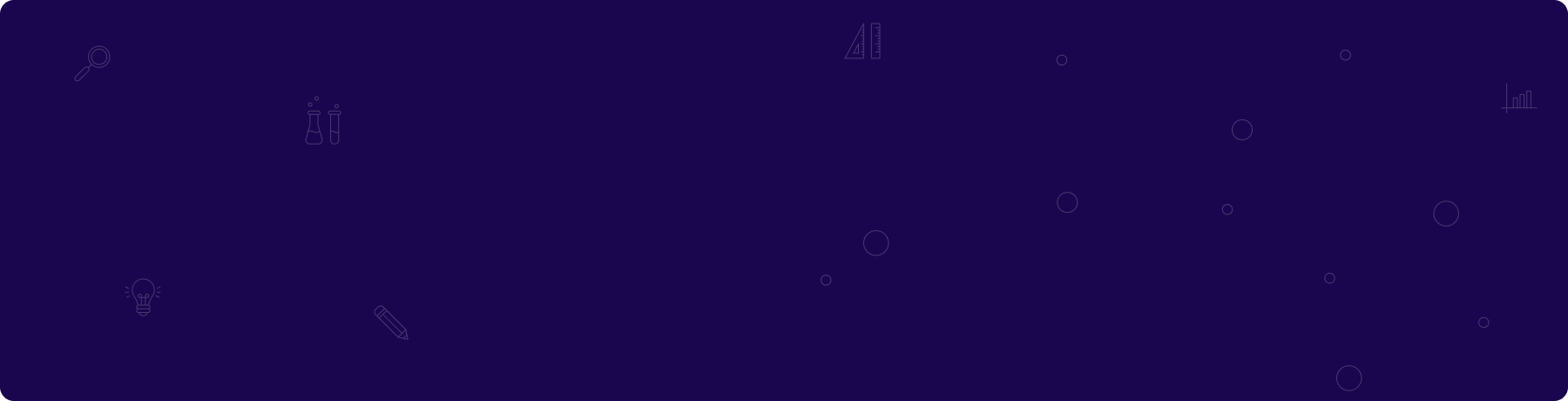
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng