Java Full stack Spring Boot and React (Inc JWT,Router,Redux)
Loại khoá học: Web Development
Mysql, MVC, CRUD, Lombok, Full Stack Development, JWT (Json Web Token)
Mô tả
In this course, we will create a new project like an online-product-seller.
When I say online-product-seller application, we can think of it like that we will have a product-list page. Somehow users or customers will see these product-lists and they can buy one of them. Of course, at the end of it, this purchase will be stored and displayed later.
And we will implement this project using Spring Boot, ReactJS, and MySQL.
In our project, we will implement CRUD operations. These CRUD operations will be for users and products. We will use users for user sign-in, sign-up and authorization operations. And we will use the products for creating, editing, and deleting product operations.
These CRUD operations will be requested from ReactJS. So on the backend, we will create an infrastructure for these CRUD operations and on the frontend, we will serve them with the user interface.
Our project goes on with User and product operations.
Our main operations will be user login, register, product-list, create-product, delete-product etc.
Also, we will go on with the role based application. So we will use different roles like “Admin”, “User”. Then we will provide different authorizations to these users according to the role.
And this all things will be provided with a secure way in both React and Spring Boot.
We will have two main components to implement our project.
These are server side and client side.
In Server Side:
Of course here, our main library will be Spring-boot. We will implement the whole infrastructure on the backend with the Spring boot. It will provide easy and fast configuration to us.
We will implement the Model view controller architecture on our project.
Spring-security will be one of the main topics in our application. Also, we will use JWT to provide security.
In Spring Boot, Data will be presented to the client as an API call so Spring Rest Controller will be used to handle it.
We will use MySQL as Database. We can use other databases also but most of us familiar with MySQL.
We will also use Object Relational Mapping with Java Persistence API and Hibernate.
You know, We can map our database tables to objects with hibernate.
We will use JPA Repository and Crud Repository in Spring Boot.
So these repository templates will handle common database operations like save, update, find, delete.
With Spring Boot, we will also use the Lombok library to clear code.
You know that we don't want to implement getter, setter, equals and hashcode. So we can escape it using Lombok @Data or @Value annotation.
We will use Maven To handle all dependencies on the server side. Actually, here we can also use Gradle. Gradle provides better performance than maven but Maven is the most common one. So we'll go on with maven.
That's all about Server side.
Let's talk about Client Side.
We will create a ReactJS application on the client side and it will provide a cool user-interface. So we will create some pages like home-page, admin dashboard, login page and register-page. Then we will assign the server apis to these pages and we will consume and produce the data from the user-interface easily and user friendly.
Last but not least, we will implement security and authorization on ReactJS also. We will work with different roles and according to these roles, we will implement unauthorized and not-found pages on the user interface also.
We will see the details of them one by one.
Bạn sẽ học được gì
Full Stack Development with React and Spring Boot
Redux State Management Application
React Router Dom Implementation on Example Cases
Json Web Token Example on Backend and Frontend
Yêu cầu
- Basic Java Knowledge
- Basic Javascript Knowledge
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
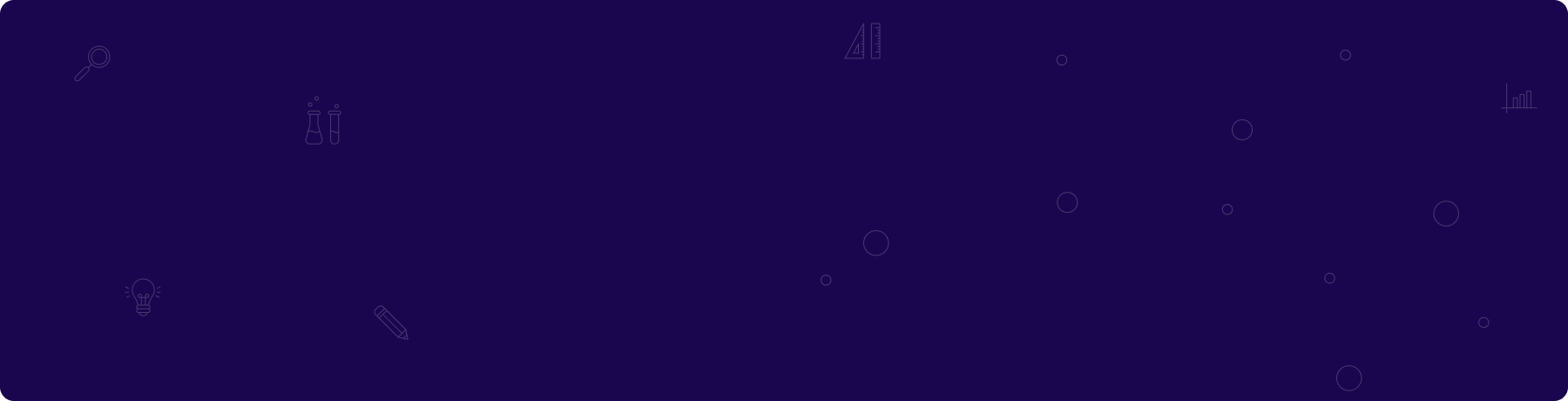
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng