Learn Advanced Modern C++
Loại khoá học: Other IT & Software
Take your knowledge of C++ to the next level!
Mô tả
This course will enhance your knowledge of the technically challenging but powerful and efficient C++ programming language.
It is designed to give you an intermediate-to-advanced level understanding of the language. There is extensive coverage of the Standard Template Library, including standard algorithm functions. Finally, a project in which you will exercise your new skills by writing a simple game.
After successfully completing this course, you should be able to apply for jobs and courses which require a good knowledge of C++.
The material is based around the modern version of the language. I teach the C++11, C++14 and C++17 standards, but also cover older variations which are still widely used.
The course is thorough and goes into the material in depth. It assumes basic C++ knowledge, such as the material in my course "Begin Programming with Modern C++": function calls, loops, conditionals and classes.
There are downloadable exercises for each video, with solutions, so you can check your understanding as you learn, gaining familiarity and confidence with the material.
I will be actively supporting the course and I will respond promptly if you have any questions or experience difficulties with the course content. Please feel free to use the Q&A feature or alternatively you can send me a private message.
Bạn sẽ học được gì
Know and understand all the important features of modern C++
Acquire a good knowledge of the Standard Template Library, including algorithms ("the best-kept secret in C++")
Learn how to use modern C++ to write code which is safer, more expressive and more efficient
Throrough coverage of C++11, C++14 and the most important features of C++17
How to write a game using Modern C++ and the SFML graphics library
Yêu cầu
- Some knowledge of C++ beyond beginner level
- A compiler which supports C++11, preferably C++14 or C++17
- Proficiency in English (B2 level, preferably C1)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
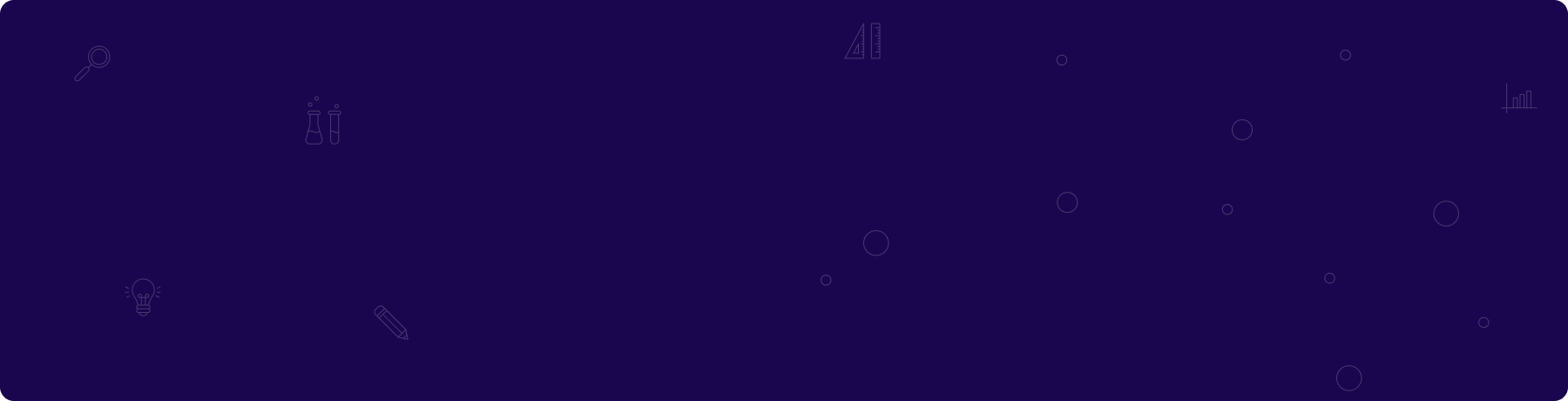
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng