Master Spring Data JPA with Hibernate: E-Commerce Project
Loại khoá học: Web Development
Learn Spring Data JPA Features and Learn to Build Domain Model Relationships for E-Commerce Project Using Hibernate ORM
Mô tả
This course supports both Spring Boot 2 and Spring Boot 3.
In this course, you will learn how to use Spring Data JPA and its features to reduce a lot of boilerplate code.
Throughout this course, we will build domain model entities (Product, ProductCategory, Order, OrderItems, User, Roles) and repositories for a simple e-commerce application.
Problem:
In typical three-layer Spring boot application architecture, we create three layers - Controller, Service, and DAO/Repository layer.
If we use JPA/Hibernate and then write a lot of coding while implementing DAO/Repository layer - We repeat the same code again and again so what will be the solution to reduce the boilerplate code?
Solution:
Spring Data JPA provides a solution to reduce a lot of boilerplate code.
We can use Spring Data JPA to reduce the amount of boilerplate code required to implement the data access object (DAO) layer.
Spring Data JPA is not a JPA provider. It is a library/framework that adds an extra layer of abstraction on top of our JPA provider (like Hibernate). Spring Data JPA uses Hibernate as a default JPA provider.
What you'll learn
Learn How to Use Spring Data JPA in the Spring Boot Application
Learn How to Use JPA Annotations to Create an Entity and Map to a Database Table
Learn 4 Types of Primary key Generation Strategies - AUTO, IDENTITY, SEQUENCE, and TABLE
Learn How to Use the Lombok Library to Reduce a Boilerplate Code
Learn Spring Data JPA Repository Interfaces and Its Hierarchy
Learn Steps to Use Spring Data JPA Repository
Learn Important Spring Data JPA Repository Methods (CRUD Operations)
Learn How to Create Query Methods or Finder Methods Using Method Names
Learn How to Create JPQL and Native SQL Queries Using @Query Annotation
Learn How to Create JPQL and Native SQL Queries With Named Queries
Learn How to Implement Pagination and Sorting Using Spring Data JPA
Learn JPA/Hibernate One-to-One Mapping Using Spring Data JPA
Learn JPA/Hibernate One to Many Mapping Using Spring Data JPA
Learn JPA/Hibernate Many to Many Mapping Using Spring Data JPA
Learn JPA Cascade Types and Fetch Types (EAGER and LAZY)
Learn building domain model relationships for e-commerce projects using Hibernate ORM framework
Learn to implement Search/Filter functionality
Learn Transaction Management with Spring Data JPA and Spring Boot
Unit Testing Spring Data JPA Repository using @DataJpaTest annotation
Tools and Technologies used
Technologies:
- Java 11+
- Spring Boot
- Spring Data JPA
- Hibernate
- Lombok
- Maven
- JUnit framework
IDE:
- IntelliJ IDEA
Database:
- MySQL database
Bạn sẽ học được gì
Learn How to Use Spring Data JPA in the Spring Boot Application
Build Domain Model Relationships for E-commerce Project Using Hibernate ORM Framework
Learn How to Use JPA Annotations to Create an Entity and Map to a Database Table
Learn 4 Types of Primary key Generation Strategies - AUTO, IDENTITY, SEQUENCE, and TABLE
Learn How to Use the Lombok Library to Reduce a Boilerplate Code
Learn Spring Data JPA Repository Interfaces and Its Hierarchy
Learn Steps to Use Spring Data JPA Repository
Learn Important Spring Data JPA Repository Methods (CRUD Operations)
Learn How to Create Query Methods or Finder Methods Using Method Names
Learn How to Create JPQL and Native SQL Queries Using @Query Annotation
Learn How to Create JPQL and Native SQL Queries With Named Queries
Learn How to Implement Pagination and Sorting Using Spring Data JPA
Learn JPA/Hibernate One to One Mapping Using Spring Data JPA
Learn JPA/Hibernate One to Many Mapping Using Spring Data JPA
Learn JPA/Hibernate Many to Many Mapping Using Spring Data JPA
Learn JPA Cascade Types and Fetch Types (EAGER and LAZY)
Learn to implement Search/Filter Functionality
Learn Transaction Management with Spring Data JPA and Spring Boot
Unit Testing Spring Data JPA Repository using @DataJpaTest annotation
Yêu cầu
- Java
- Good to know Spring Boot Basics
- Basic Understanding of JPA and Hibernate
- Basic Understanding of SQL
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
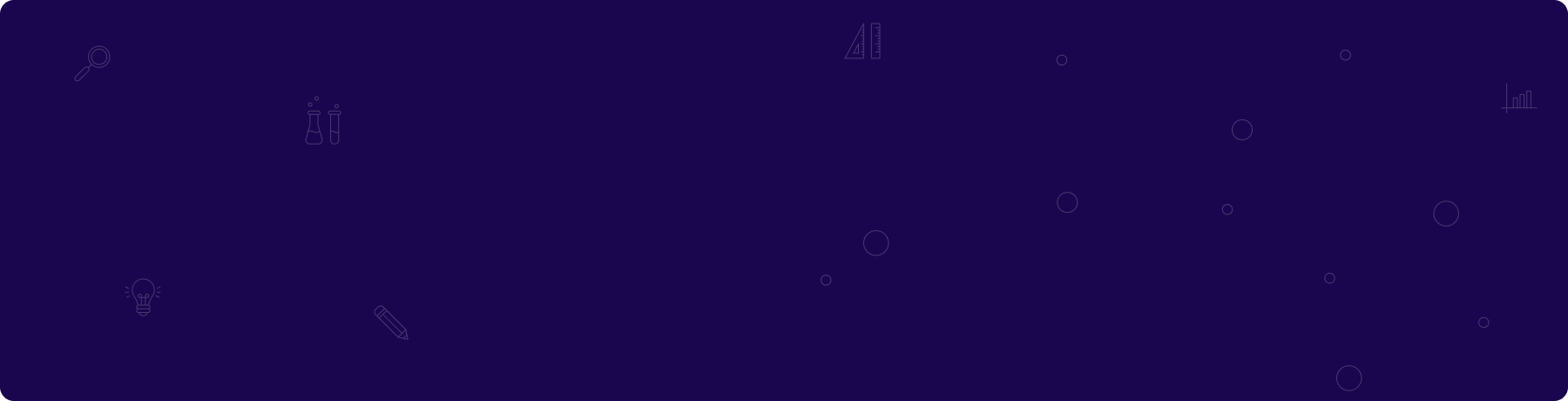
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng