Data Structures & Algorithms in Java + 130 Leetcode Problems
Loại khoá học: Programming Languages
Mastering Leetcode In Java - Step By Step
Mô tả
The "Mastering the Top 100 Leetcode Problems" course is a comprehensive training program designed to help you excel in coding interviews by focusing on the top 100 Leetcode problems.
Leetcode is a well-known platform that offers a vast collection of coding challenges frequently used by tech companies during their hiring process.
In this course, we will tackle the most frequently encountered problems in coding interviews.
Each problem will be thoroughly analyzed, providing you with valuable insights into the underlying concepts and problem-solving techniques.
You will learn how to approach problems systematically, break them down into smaller manageable tasks, and devise efficient algorithms to solve them.
A key aspect of this course is the live implementation of code.
Each problem will be demonstrated in real-time, allowing you to witness the coding process firsthand.
This practical approach will help solidify your understanding and improve your coding skills.
You will gain insights into efficient coding practices, optimization techniques, and common pitfalls to avoid.
We will go over each of the problems in extreme detail, going through the thought process, and live implementation for the code.
To support your learning journey, the course will provide code sample files accompanying the video lectures.
These resources will serve as valuable references and guides, assisting you in implementing the solutions effectively.
Bạn sẽ học được gì
Yêu cầu
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
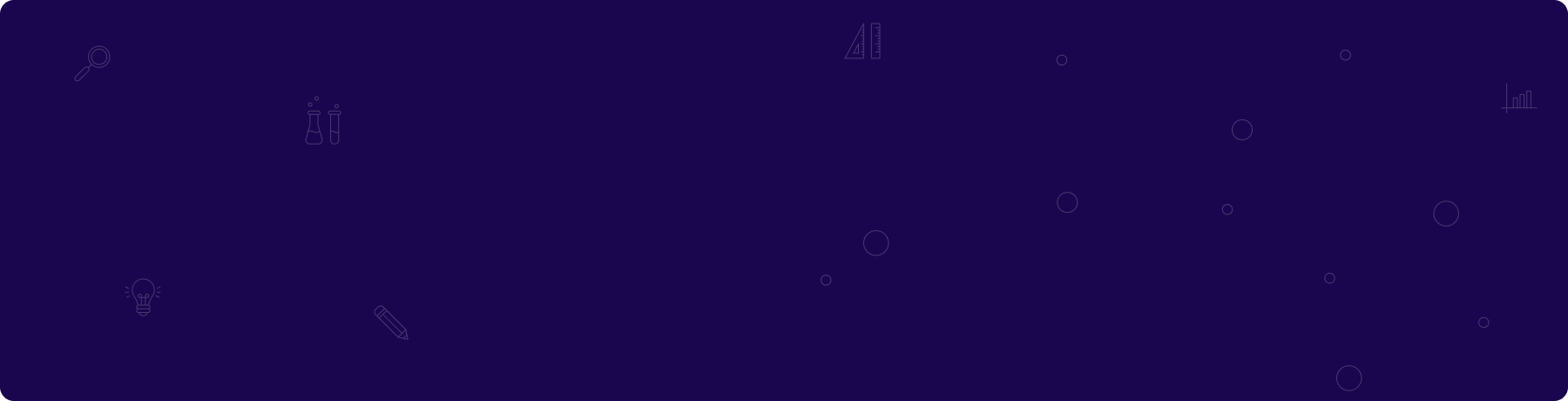
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng