Mastering webRTC - real-time video and screen-share
Loại khoá học: Web Development
Working w/MediaStreams, the mic, camera, & screen. Connecting the browsers - PeerConnection & Signaling, w/React
Mô tả
TL;DR - you'll learn WebRTC in this course - how to get a video feed and setup a video chat between browsers with just JavaScript. The first section and a few later projects are front-end only, the connection section and later projects require Node.js and Socketio.
It's 2023. Whether because of the pandemic, chat bots, or cost savings, working remote is a thing. Telemedicine is a thing. Talking to people 6 time zones away is a thing. You can abstract your users from your app to Zoom because it always works, but you lose all control and tracking of the interaction.
Enter webRTC.
WebRTC is one of the browsers must mind blowing apis. It allows access to the mic, camera, even the screen AND to share them across a network socket DIRECTLY to another browser. No server (mostly) or other middleman to add bandwidth, bugs, and chaos.
Along with websockets, webRTC presents the video-side of browser real-time communication, bridging one of the last gaps in both human and web-based communication. There's a good chance if you're reading this, you've heard about webRTC. Maybe even done a tutorial on it. But how far did you get? In my experience, the vast majority of the material on the web goes no farther than a quick-start, zoom clone. You don't learn how anything works, never look at the docs, and are stuck at the end wondering what to do now. Is that all webRTC can do? The remaining shred of material is waaaaay over everyone's head. The fact that the webRTC was released about the same time as the websocket API and most developers still don't know how to use it is evidence of the gap.
This course is the first step to alleviate that! It is not a quick start guide to webRTC. There are loads of those all over the Internet. You should definitely look elsewhere if you are wanting a 10 minute intro to the 3-4 things you need to know to make a basic zoom clone. On the other hand, if you are looking to really learn one of the most awesome JavaScript APIs that no one seems to know, you should stick around. Like Express and other JavaScript/Node pieces, it's getting passed over in the wave to learn just enough to get to the term "full-stack developer." My main goal is to help you figure out how to go from being a good developer to a great developer. Understanding... not just knowing a few methods... WebRTC is part of that!
I first used webRTC in 2015 for a startup similar to telemedicine. I've been following since and have been frustrated that it hasn't gotten more mainstream notice due to Apple's reluctant support, but mostly because devs don't know it. It opens the way for so many improvements to existing applications and obvious groundwork for new ones. Let's change that :) Prepare to for a detailed look at webRTC and start going in-app real-time video/screen chat.
What we cover:
Front-end only portion
getUserMedia() - getting access to the mic and camera in the browser
playing the feed in a <video />
MediaStream and MediaStreamTrack - what makes up a video feed
Constraints - getSupportedConstraints() and getCapabilities() - seeing what this browser can do
applyConstraints - changing the feed on the fly
Recording video/audio and playing it back
Capturing the Screen for screen share and recording it
Changing input/output devices in your feed
Back-End required (node, socketio)
RTCPeerConnection - the heart of webRTC
Creating an offer and an answer
Setting RTCSessionDescription
The Signaling Process
Build a signaling server with socketio
Gathering ICE Candidates
Building a React app w/redux that uses webRTC
Bạn sẽ học được gì
Connect two or more computers audio/video streams
Set up a signaling server to act as a middle man to negotiate the connection
How to integrate webRTC into a React App w/redux
Code organization to minimize confusion as your app grows
Manage 4 (yes 4) different async sources to create an incredible web app
Yêu cầu
- Need to be familiar with getUserMedia() or have taken part 1
- Experience with JavaScript and Node (not a ninja, but decent exposure)
- We use socketio to build our signaling server. You must know it, or be able to follow me
- For later sections: Access to a Linux machine (like AWS, Azure, DigitalOcean, etc)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
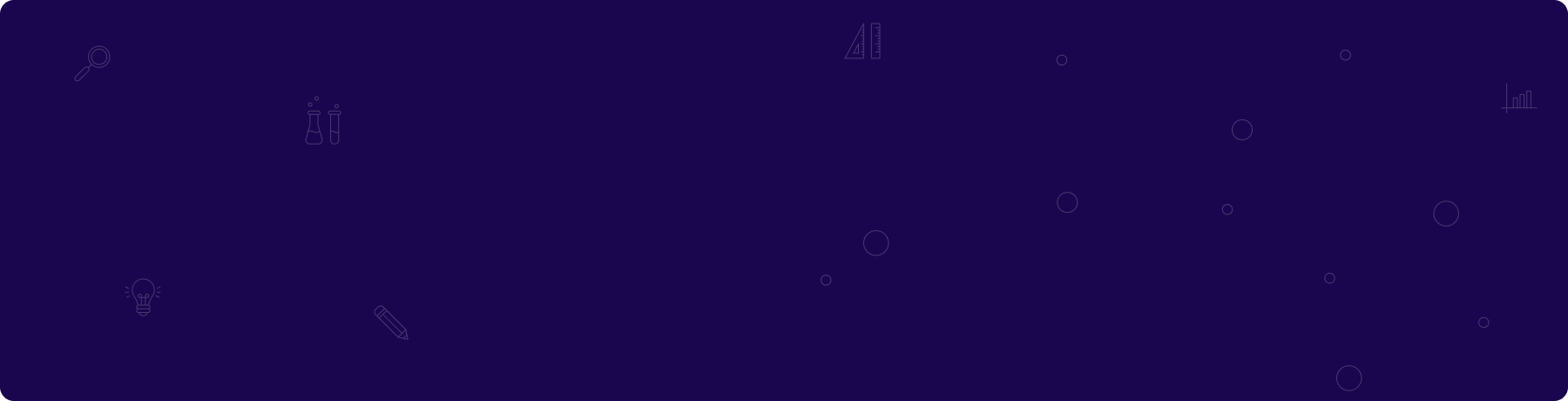
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng