Modern JavaScript (Complete guide, from Novice to Ninja)
Loại khoá học: Programming Languages
Learn Modern JavaScript from the very start to ninja-level & build awesome JavaScript applications.
Mô tả
Hey gang, and welcome to your first step on the path to becoming a JavaScript ninja! In this course I'll be teaching you my absolute favourite language (JavaScript!) from the very beginning, right through to creating fully-fledged, dynamic & interactive web experiences.
We'll cover all the basics to get you up-and-running quickly, before diving in to some of the really fun stuff like web-page manipulation, creating interactive forms, popups & other cool effects. Along the way we'll be using the latest additions to the JavaScript specification (ES6, 7 & beyond) and maintaining good coding standards to keep our code clean and effective!
Once we master the basics, we'll dive into several real-life JavaScript projects, including an interactive quiz, a weather app, a real-time chat application and a small UI library you can use in all your future projects!
We'll also take a look at some more advanced topics - object oriented programming, asynchronous code, real-time databases using Firebase (including a new chapter about Firebase 9) and much more. Finally, we'll be setting up a modern work-flow using Webpack & Babel, so that by the end of this course you'll be no less than a black-belt JavaScript developer with a lot of coding techniques in your tool-belt.
Speaking of ninjas, I'm also known as The Net Ninja on YouTube, where you'll find hundreds of free coding tutorials, so feel free to pop by to say hello :).
Bạn sẽ học được gì
Learn how to program with modern JavaScript, from the very beginning to more advanced topics
Learn all about OOP (object-oriented programming) with JavaScript, working with prototypes & classes
Learn how to create real-world front-end applications with JavaScript (quizes, weather apps, chat rooms etc)
Learn how to make useful JavaScript driven UI components like popups, drop-downs, tabs, tool-tips & more.
Learn how to use modern, cutting-edge JavaScript features today by using a modern workflow (Babel & Webpack)
Learn how to use real-time databases to store, retrieve and update application data
Explore API's to make the most of third-party data (such as weather information)
Yêu cầu
- A basic grasp of HTML & CSS (how to create simple, static web pages)
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
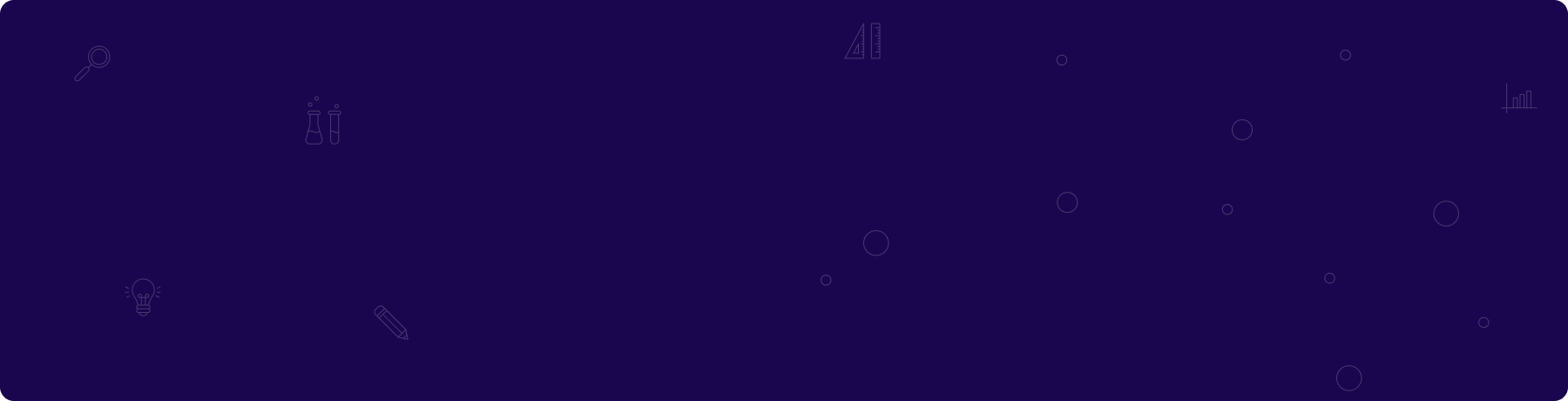
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng