Next JS & Typescript with Shopify Integration - Full Guide
Loại khoá học: Web Development
Learn modern Next JS(Next 10+). Code everything in Typescript and integrate with Shopify. Professional app architecture.
Mô tả
What is Next.js?
Next.js is a React-based framework that provides infrastructure and simple development experience for server-side rendered(SSR) & static page applications.
An intuitive page-based routing system (with support for dynamic routes)
Pre-rendering, both static generation (SSG) and server-side rendering (SSR) are supported on a per-page basis
What is Typescript?
TypeScript is an open-source language which builds on JavaScript, one of the world’s most used tools, by adding static type definitions.
Is this course right for you?
If you plan to start your career as a developer or improve your programming skills, this course is right for you. Learn how to build a professional web application inspired by the project of Next JS developers.
Start with learning the Typescript language and progress into building your own e-commerce application.
This resource is the only thing you need to start Web Development with Next.js. During this course, you will get the confidence and skills required to start your own projects. In addition, you will get the right mindset to apply for a developer career and improve in modern frameworks like Next.js and React.js.
What are you going to work on?
You will build an e-commerce application from scratch. You will learn how to write code in Typescript language, a superset of Javascript providing additional features, and a static type checker.
The course starts with an explanation and practical examples of the Typescript language. The typescript section is optional but it explains a lot of types of structures that will be used throughout the course.
After typescript lectures, you will start building your e-commerce app. You will learn how to structure the application in a modular and clean way.
You will build your own React components from the scratch. As the styling framework, the course is utilizing PostCSS and Tailwind CSS which is a great choice for every project.
Later in the course, you will learn the latest patterns on how to use hook functions efficiently. Entire checkout functionality is built on top of the hook functions. Hooks are reactive, modular, and easy to get tested.
At the end of the course, we will hook up the application with Shopify, and we will deploy it to the Vercel platform so anyone on the internet can access it.
Bạn sẽ học được gì
Build a modern, well-architectured app from scratch
Get most of the JS with Typescript
Create applications with real use case
Create Shopify integration
Yêu cầu
- Basic Javascript knowledge is required
- Fundamentals in React, HTML and CSS
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
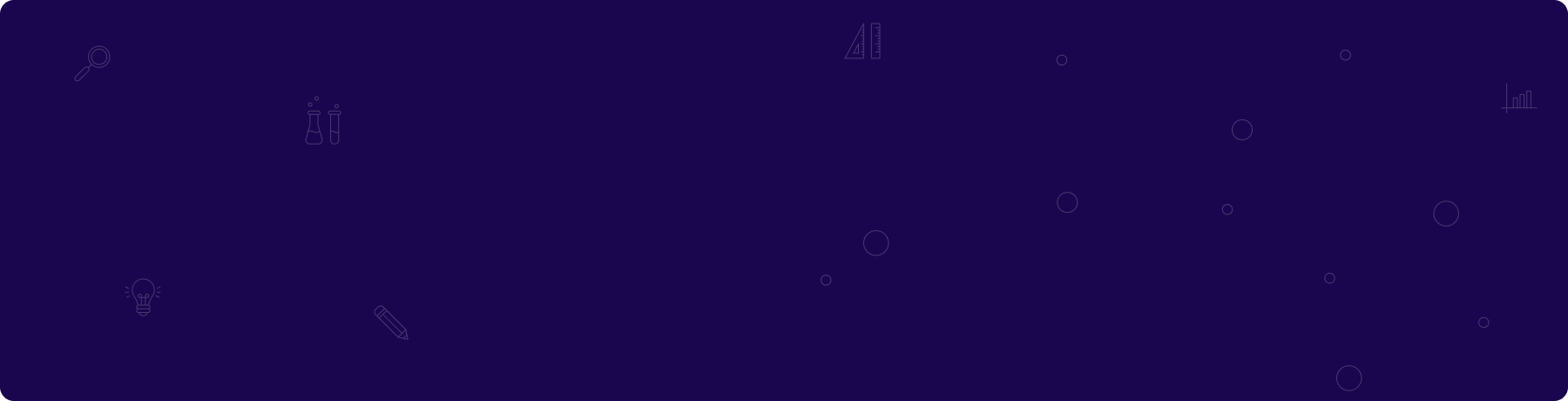
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng