React Front To Back
Loại khoá học: Web Development
Learn modern React by building 4 projects including a Firebase 9 app and a full stack MERN app
Mô tả
This course is for anyone that wants to learn React and also for React developers looking to build some projects and sharpen their skills.
The first project (Feedback App) is structured in a way so I can explain the fundamentals such as components, hooks, props, state, etc in a way that beginners can understand. The second project (Github Finder) will show you how to work with 3rd party APIs and the third project (House Marketplace) is a larger app that uses Firebase 9 and includes authentication, Firestore queries, file storage and more.
The final project has been added! It is a fullstack MERN support ticket system that uses Redux and Redux Toolkit.
Here are some of the things you will learn in this course:
React fundamentals including components, props, hooks, state, etc
React hooks such as useState, useEffect, useContext, useReducer, useRef, etc
Creating custom hooks
React Router v6 (latest version)
How to handle global state with context, reducers and hooks
Firebase 9 authentication, queries, storage
Deploying React apps to Vercel & Netlify
Basic Animation with Framer Motion
Implement Leaflet maps and Swiper sliders
Fullstack MERN (MongoDB, Express, React, Node.js)
REST API creation
Redux
Redux Toolkit
Authentication with JWT
Much More...
This course has been completely re-done with new projects and concepts from the old course. I have a few React courses and this is the one that I always suggest people start with before moving on to the MERN courses
Bạn sẽ học được gì
Learn modern React by building 4 projects
Suitable For Both Beginners & Intermediate React Developers
Feedback app with in depth explanation of React fundamentals
Build a house marketplace with React and Firebase 9
Learn React hooks and how to create custom hooks
Learn how to use context and reducers to manage global state
Build a Fullstack MERN support ticket system with Redux Toolkit
Yêu cầu
- You should know JavasScript pretty well before learning React or any framework
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
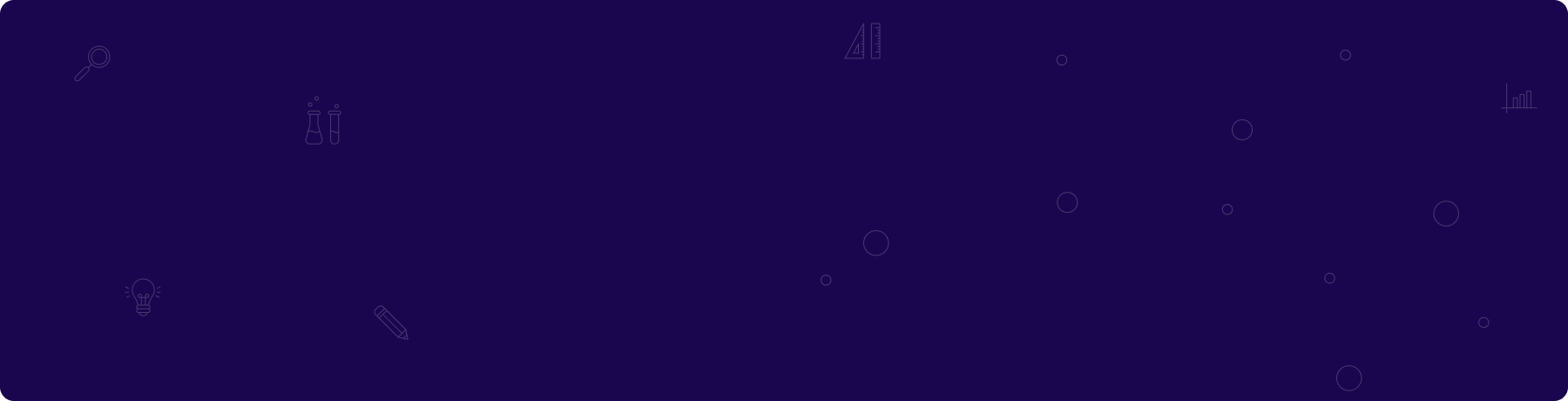
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng