React js. From the beginning. w/ Redux and React Router
Loại khoá học: Web Development
Learn React from the beginning! Including React Router, redux, hooks, context, front-end Stripe & a big project
Mô tả
Important note: This course was made in 2017 when classes were the primary model for React and ES6 was new. Since then, the model has changed substantially to focus on functional programming (hooks). Smaller, but important changes like using next.js, redux-tool-kit, etc. have also gotten traction. I do cover hooks in both React and Redux (react-redux module), but the course is focused around the older model. It still works, it's just "outdated" as current React goes. Please be aware of that if you are new, and if you prefer a class based model, then this might be exactly what you are looking for.
This course:
Starting with Angular 1 in 2010, JavaScript frameworks exploded onto the scene and are required by virtually every large website on the Internet. React, which appeared a few years later in 2013 has become the dominant tool in that group. What does that mean for you? You can learn to use the same front-end framework used by Facebook, Amazon, Netflix, BBC, Airbnb, and Ebay, to name a few. And you won't just learn how to use it, but you'll learn the fundamentals around it.
TL;DR - React is awesome. I've been teaching it professionally for 8 years and love it. Learn it here!
What I need from you:
I need you to know JavaScript, CSS, and HTML.
It's not required to use React, but React makes very heavy use of ES6 (the 2015 update to JavaScript), so the course will use ES6 after the first main section. I will briefly mention how it works as needed, and add a supplemental section that covers those parts.
I need you know how to navigate your computer, not be afraid of the terminal, and ask questions as you have them!
Why me:
I first used React in 2014 for a small company website. I've also used Angular, Vue, and Ember along with most other major UI frameworks and I'm a believer in React. I've taught it professionally for 4 years, and have built sites for some of the largest companies in the United States using React Router and redux alongside. I will teach you one step at a time, whiteboard, and start from ground zero before getting to webpack and eventually Redux. Prepare to start learning the best JavaScript framework!
The sections in bold will be finished no later than August 15. I will send out a message when they are complete.
Sections:
Environment Setup (skip if you have node installed already)
React 101
State and Events
The Component Lifecycle and HTTP
Project 1 - Flash Card app (AWS services)
React Router
Redux
Redux Middleware (redux-promise and redux-thunk)
AirBnB Clone with Redux
Overview of Auth From a Front-end Perspective
Building/Preparing React for Deployment
Hooks - the "2020 way" to do React
Context
Supplemental - ES6 for React
Bạn sẽ học được gì
How to use React to make awesome front-end UIs!
How to set up, navigate, and use create-react-app to build React applications with ease
How to use React without webpack or ES6
The React component lifecycle system
Have a good idea how to make a large project (we do a small clone of AirBnB)
Yêu cầu
- Familiarity with JavaScript. I don't expect you to be a JS jedi, but you should not be new
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
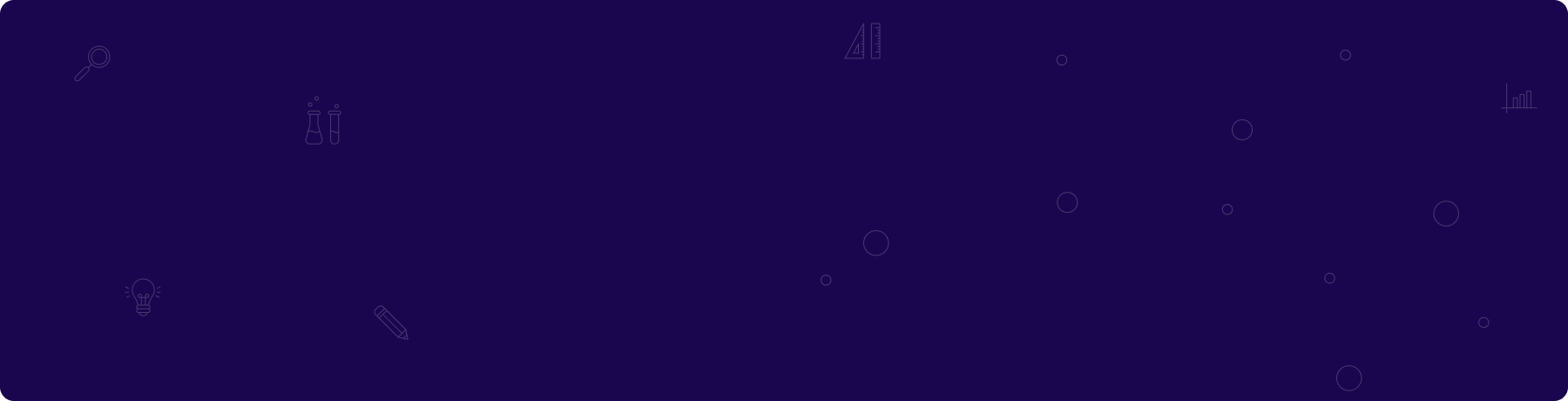
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng