Real-World TypeScript Unit Testing
Loại khoá học: Web Development
Create unit tests for real world typescript system with few modules using vitest or jest
Mô tả
You’re here because you know typescript and want to write better code with fewer bugs using unit tests.
I am here because I can help you accomplish your mission. I have B.s.c + M.s.c in mechanical engineering. I have been making software applications for over 20 years in the Hi-Tec industry and have much teaching experience. You can trust me and my teaching methods. I have learned many technologies in the past (check my LinkedIn profile), and I know exactly what is needed to learn and how.
In this course, I teach you everything you need about unit tests, and I use a real-world system, typescript, vitest, and jest.
The task of writing a unit test is not simple because there are many moving parts:
- Unit test of logic code
- Unit test of code with side effects like accessing the web
- Unit test of a module that uses another module
- Mocks
- Timers
- Unit test of UI
- Unit test of code that runs on the server
- Jsdom, testing library, react testing library
- Some tools like jest need a nontrivial setup to work with typescript and ES module
- New competitor to jest, e.g., vitest
- Coverage test
This ocean is very hard to swim without first learning it properly, and this is done in this course.
Learning in this course is not just video lessons; there are other important learning materials that most courses do not provide as a whole :
- Quiz after EVERY lesson
- Coding exercise at the end of EVERY coding section
- pdf file with all the lectures
- pdf file with a dictionary of all the material that I teach in this course
Bạn sẽ học được gì
Create unit tests for real world typescript client and or server applications using vitest or jest
Write better typescript code with less bugs
Ship code to production quickly and confidently
Represent your system using advanced diagrams : block diagram ,UML sequence diagram and UML class diagram
Write unit testing of pure logic code using test and expect
Invoke test coverage tool like istanbul
Write unit testing for code with side effect using spyOn , fn and mock
Use advanced concepts for unit testing : refactoring , debugging , filtering
Use isolated test and sociable test for unit test with module interaction
Create unit testing involving real and fake timer such as in sinonjs/fake-timers
Create unit testing for frontend vanilla UI using jsdom : document , querySelector
Create unit testing for frontend vanilla UI using dom testing library : getByText , getByRole , waitFor
Create unit testing for frontend react UI using react testing library : render , screen
Use advanced typescript for better code : union , enum , type any and unknown , polymorphism using inheritance , class diagram
Create unit tests using vitest and jest and compare between these tools
Yêu cầu
- Knowledge of typescript is required
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
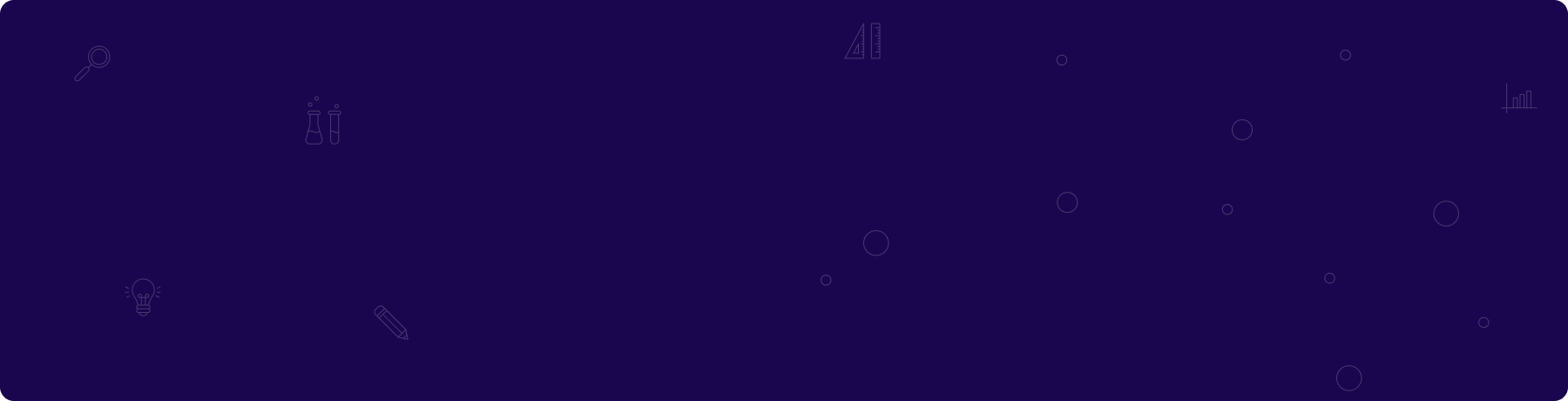
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng