The Modern React Bootcamp (Hooks, Context, NextJS, Router)
Loại khoá học: Web Development
Just published! Follow the same curriculum I teach my students in SF. 10+ projects including one HUGE application!
Mô tả
EXPANDED and UPDATED to include new sections on Next JS and Server-Side Rendering!
Welcome to the best online resource for learning React! Published in April 2019, this course is brand new and covers the latest in React. This course follows the exact same React curriculum my in-person bootcamp students follow in San Francisco, where students have gone on to get jobs at places like Google, Apple, Pinterest, & Linkedin. This is the most polished React course online, and it's the only course that is based on REAL bootcamp curriculum that has been tried and tested in the classroom.
This course builds up concepts one at a time, cementing each new topic with an expertly designed exercise or project to test your knowledge. It includes a huge variety of beautiful exercises, projects, and games that we create together from scratch. Sometimes we mix things up and give you a broken React app and ask you to fix it or optimize the code. Check out the promo video to see a couple of the course exercises. The course culminates in one huge capstone project, which is the largest project I've ever built for any of my online courses. I'm really excited about it :)
React is the most popular JS library around, and there's never been a better time to learn it! Companies all over the world are turning to React to help manage their JavaScript chaos, including tech giants like Facebook, Airbnb, and Uber. React is consistently voted the most loved and most wanted front-end framework by developers, and it's clear why! React is a joy to use, and it makes writing maintainable and modular JavaScript code a breeze.
If you're new to frameworks, React is the ideal first framework to learn. It's easy enough to learn the basics, but it doesn't teach you bad habits. Even if you've already enrolled in another React course, this course is worth your time for the exercises and projects alone! This curriculum is the product of two years of development and iteration in the bootcamp classroom. All the lectures, exercises, and projects have been tweaked and improved based on real student feedback. You won't find anything else online as structured and polished as this course.
The highlights:
Learn React, from the very basics up to advanced topics like Next JS, React Router, Higher Order Components and Hooks and the Context API.
Build one massive capstone application, complete with drag & drop, animations, route transitions, complex form validations, and more.
Learn the latest in React, including Hooks (my favorite part of React!). We build a complete app using Hooks, including multiple custom hooks.
Learn state management using the useContext and useReducer hooks, to mimic some of the functionality of Redux.
You get tons of detailed handouts and cheatsheets that you can refer back to whenever you need to. Think of this as a React textbook you can study at any point if you get tired of videos.
What you get:
250+ videos
Slick, interactive slide decks custom made for each section
Detailed handouts and cheatsheets, the React "textbook".
Dozens of exercises, projects, and quizzes.
One massive code-along project with nearly 20 different React components.
Free Webpack Mini Course!
Bạn sẽ học được gì
React Hooks! (My favorite part of React!)
The new Context API
State management w/ useReducer + use Context (Redux Lite)
The basics of React (props, state, etc)
Master React Router
Build tons of projects, each with a beautiful interface
React State Management Patterns
Drag & Drop With React
Writing dynamically styled components w/ JSS
Common React Router Patterns
Work with tons of libraries and tools
Integrate UI libraries like Material UI and Bootstrap into your React apps
React Design Patterns and Strategies
Learn the ins and outs of JSS!
Learn how to easily use React to build responsive apps
Add complex animations to React projects
Debug and Fix buggy React code
Optimize React components
Integrate React with APIs
Learn the basics of Webpack in a free mini-course!
DOM events in React
Forms and complex validations in React
Using Context API w/ Hooks
Yêu cầu
- You'll need some experience with HTML, CSS, and JavaScript, but you don't need to be an expert.
- You don't need advanced JavaScript knowledge. ES7 features are explained in the course as we go.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
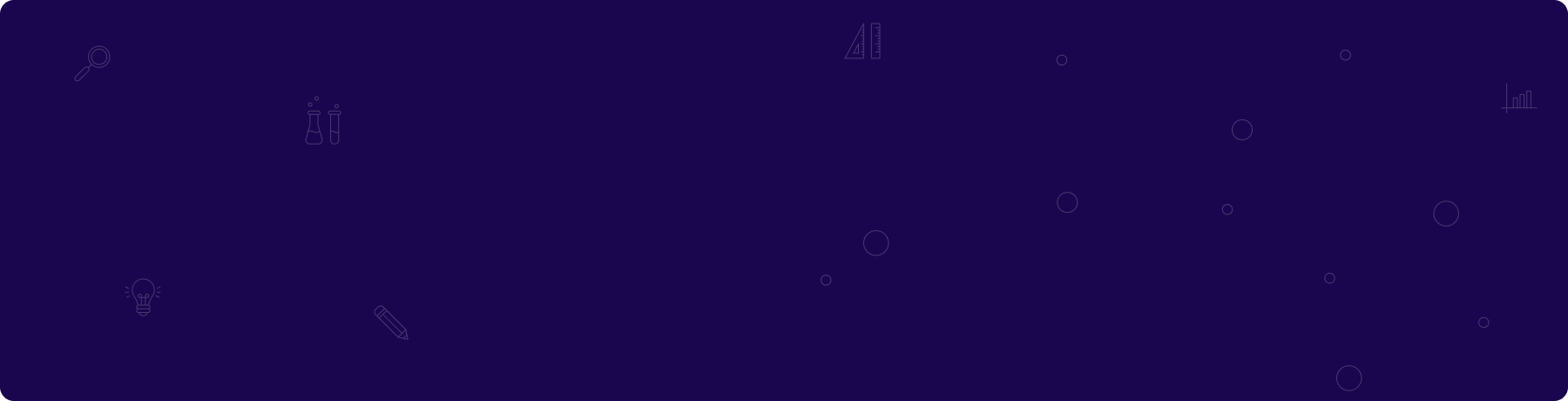
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng