The Next.js 13 Bootcamp - The Complete Developer Guide
Loại khoá học: Web Development
Learn all the new features of Next.js 13 by building a restaurant reservation app.
Mô tả
NextJS 13 is an amazing higher level framework, built on top of React, that will totally change the way you think about and build web applications.
In this course, we will learn all about the amazing features NextJS 13 has to offer by building a restaurant reservation application where people can view restaurants and reserve tables.
Firstly we are going to explore the file system in NextJS 13. We are going to learn how to create static and dynamic routes by simply defining files and folders.
We are then going to look at how to handle loading, error, not found, and success states within our application.
Then we will move onto the rendering modes of React components. We will learn about the differences between server and client components and when we should use one over the other.
Following that, we will dive into the server side of NextJS. We will spin up a Postgres database and define the models we need with Prisma (ORM). We will learn how to fetch data from this database, depending on the component we are utilizing.
We will then move to the backend and start defining some authentication endpoints. We will implement authentication for scratch by utilizing middleware, hashers and json web tokens.
The authentication endpoints will be consumed by our client and the user data will be stored globally with the context API.
We then move onto the completing our application by building the availability and scheduling system. We will utilize multiple algorithms and logical thinking to accomplish this.
Bạn sẽ học được gì
Build a large production ready application with Next 13
Handle loading, error, success, and not found states appropriately
The different rendering modes of React components
Handle authentication with middleware and server routes
Yêu cầu
- You will need to know the basics of ReactJS. A good understanding of hooks and components would suffice.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
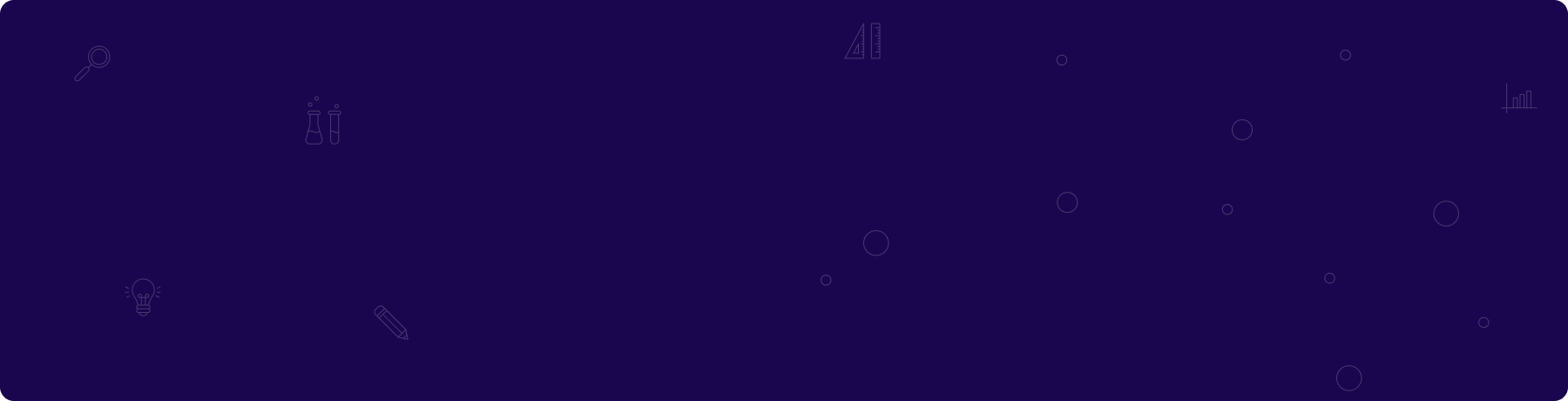
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng