The Swift Arcade Data Structures and Algorithms Bootcamp
Loại khoá học: Mobile Development
How to ace your Silicon Valley style coding interview
Mô tả
This course is about getting you up-to-speed quickly on the fundamental computer science concepts you are going to be expected to know if you want interview at any large Silicon Valley tech company (Google, Apple, Facebook, Amazon, or Spotify).
Topics include
Arrays
Linked Lists
Big O notation
Stacks & Queues
Hash Tables
Binary Trees
Dynamic Programming & Memoization
Bubble Sort / Merge Sort / Quick Sort
Graphs
Breadth First Search
Depth First Search
More...
What you get
With this course you get
Over 115 beautifully hand crafted HD videos walking you through every aspect of how all these data structures and algorithms work
Practices questions and personal walkthroughs of the most commonly asked interview questions
My personal notes on interviews I have personally had with Spotify, Facebook, Amazon, and others
A section called The Classics where we walk through classic interview questions no interviewee should be with out
Interview tips on soft skills big tech companies look for when hiring and techniques on how to answer
What you save
By investing in yourself with this course you are saving yourself the most precious thing you’ve got - time. I have spent a year scouring the web looking for the best examples, the simplest explanations, the best visualizations on how to explain how this stuff works, and assembled it all into one, quick, easy to digest place.
Let's do this together
Learning data structures and algorithms doesn’t have to be a chore. It can be fun. And I want you to know I am here for you every step of the way. Ask me any question. I usually get back to my students with 24 hrs. And together, we will get you the understanding behind how these things work.
I also don’t have a formal computer science background
Look. I know what it’s like not to know how this stuff works. And, like you, I have had to learn this stuff from scratch.
But I am here to tell you it can be done. I have no formal computer science background. I am not classically trained as a computer scientist. But by learning this material, I landed my dream job as an engineer at Spotify in San Francisco. And so can you.
So what are you waiting for? Sign up and get started on your journey today.
Testimonials
This is the best course I ever had, very organized, clear explaining and easy to understand topics. The important thing, I was able to pass and solve, the coding interview as iOS developer, after taking this course. Many thanks Jonathan.
Thank you for this amazing course. I have been developing iOS for almost 7 years now. honestly I didn't know about 90% of the topics that you are covering in this course. Thank You!
The instructor is valid, truly humble and fun. It's been a pleasure to follow this course.
I am leaving a 5 star here because not only does this course expose you to Algorithms and DataStructures, it builds your confidence for any interview and you learn that we are all human and can't always be perfect with our approach. Had two Algorithms & DataStructure interview with two big techs and solved passed the Stage.
By the time I had taken this course I had already built my first app "janet." and had it launched on the App Store. After the launch I started looking for an iOS developer position at a tech company. Come to find out that although I had cloned dozens of different kinds of popular apps and successfully launching my own, I didn't know the things I needed in order to get a job as a developer. After getting a few books, taking a few courses on swift data structures and getting through to the last round of the Facebook interviews, I found this course. After completing this course over a weekend, I started crushing coding challenges and really understood the code that I was writing. Not even a month later I landed my first iOS Engineering position! Jonathan has been the best instructor I have found for iOS on Udemy. I just purchased his new course Professional iOS Development and I can't wait to go through it! Thanks for everything so far, Jonathan!
This course has been fantastic for filling in the gaps in my programming knowledge! I am feeling much more confident about answering questions in a tech interview now!
Amazing course, worth taking even if you are intermediate/advanced and want to refresh concepts. The instructor is phenomenal!! Thank you so much for making this course!!
Bạn sẽ học được gì
How to pass technical interviews at large tech companies
Knowledge and mastery of data structures and algorithms
How to answer the most commonly asked interview questions
How to interview confidently and well
Land your dream job
Yêu cầu
- Basic knowledge of Swift programming language
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
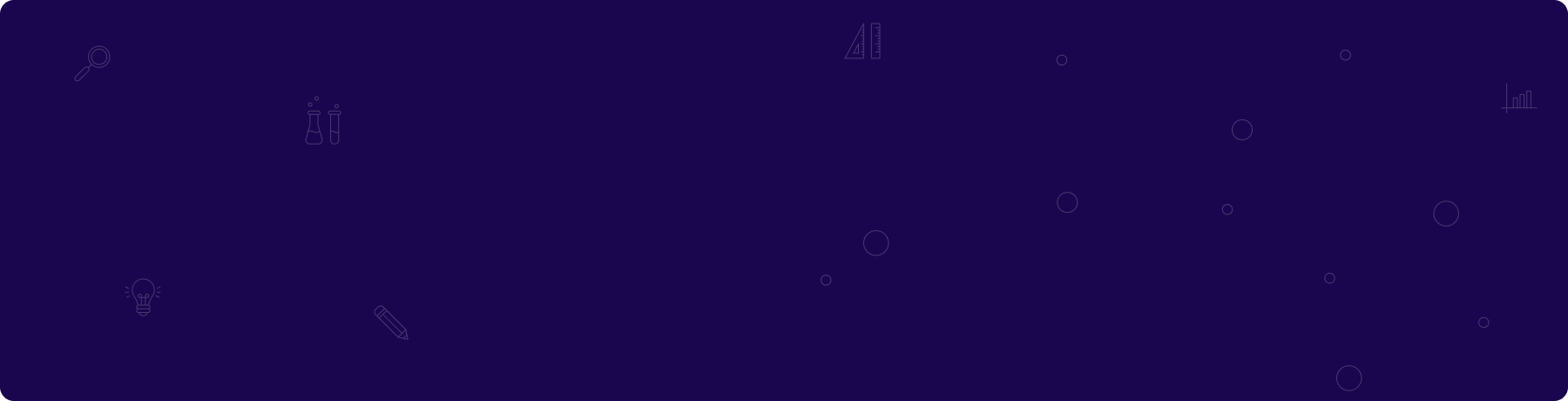
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng