NodeJS Tutorial and Projects Course
Loại khoá học: Web Development
Learn Node.js by building real-world applications with Node JS, Express, MongoDB.
Mô tả
Welcome to NodeJS Tutorial and Projects Course.
I guess let’s start by answering the most pressing question first. What is a NodeJS? And even though there are plenty of good answers out there the one that I like the most is this one - "NodeJS is an environment to run Javascript outside of the Browser". NodeJS was created in 2009 and it's built on top of Chrome's V8 Javascript Engine. As you are probably aware of, every browser has an engine, a tool that compiles our code down to machine code and Chrome uses one by the name of V8. Since the moment it was created, Node has evolved tremendously, and while there are many things to like about Node, some of the main ones are - large community, since that tremendously saves time on feature development, as well as the fact that with the help of Node, it's never been easier to build Full-Stack Apps, since both Front-End And Back-End are built in one language, and you guessed that language is our beloved Javascript.
During the course we will cover following main technologies - NodeJS, ExpressJS, MongoDB, Mongoose, JWT and many smaller ones as well.
Course consists of
- Node Tutorial
- Express Tutorial
- Projects
- Task Manager API
- Store API
- JWT Basics
- Jobs API
- File Upload
- Send Email
- Stripe Payment
- E-Commerce API
- Email Workflow
Bạn sẽ học được gì
Make Great Projects Node and Express
Yêu cầu
- Basic Knowledge of HTML, CSS, JS (ES6) is Required.
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
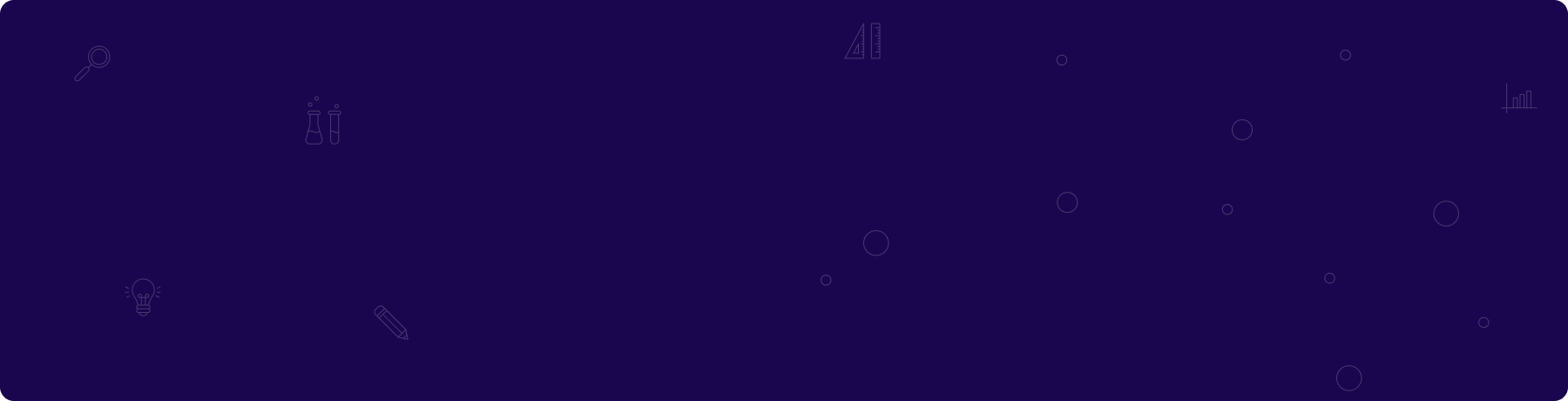
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng