React Testing with Jest and Enzyme
Loại khoá học: Web Development
Improve your React, Redux, Hooks and Context Code with Test Driven Development
Mô tả
Please read the first lecture (reproduced at the end of this course description) before deciding to purchase this course.
In short, Enzyme is no longer actively supported. Unless you're interested in learning Enzyme specifically (because you're working on a legacy React project that uses Enzyme tests), I recommend taking a React Testing Library course instead.
**************************************************************************************************************
Take your React code to the next level by learning Test Driven Development (TDD) with Jest and Enzyme! Jest is a powerful, flexible testing framework, and Enzyme provides tools to test React and Redux applications. In this course, you will learn to test:
React hooks, including useEffect, useState and useReducer
Asynchronous functions using Axios
Redux action creators and reducers
Complex Redux action creators that use Redux Thunk
React context, including context with embedded state
You will also learn how to make the most of Jest capabilities, including
Mocking and restoring individual properties of modules
Mocking entire modules
Controlling which tests run using, .skip, and .only
Running test suites multiple times with different data, using .each
Please Note: This course does not cover Jest snapshots, as they are not compatible with the TDD mode of testing.
Why Learn to Test?
Test Driven Development will help you write better organized code that’s easier to maintain, which will save you time in the long run. Your tests provide value to your software development team, since others know they can rely on your code. Employers want developers with testing skills!
Unit tests and Functional Tests
This course focuses on unit tests. Unit tests are tightly coupled to specific areas of code, which leads to easy diagnosis of failures, and a great match for Test-Driven Development. The course also teaches functional tests, which are modeled on user flows (and resulting behavior from the user perspective). Functional tests are not connected to code, which makes them more difficult to diagnose, but more resilient to code refactors.
Learn the Reasons behind the Syntax
This course discusses tradeoffs when considering different approaches to testing, leaving you confident in the testing choices you make. Furthermore, you will deepen your understanding of React, Redux and Context as we dig into how and why we test each aspect.
Practice your New Skills
You will also have opportunities to practice what you’ve learned. There are occasional “quizzes” while we’re building the course projects, where you can apply what you learned, and then watch a video to see the solution. There are also two sets of challenges to extend the course projects, with solutions on GitHub.
**************************************************************************************************************
**************************************************************************************************************
This lecture/article is reproduced here, since Udemy articles do not preview well. Unfortunately, you will not get the full experience, as links are not available in the course description.
First lecture / article: The Author's Thoughts on Enzyme and React 18
The stark reality: Enzyme is not compatible with React 18, and probably never will be.
The History
I started writing this course in March 2018, and it was published in May 2018. At that time, Enzyme was the primary option for testing React components with Jest. React Testing Library (Enzyme's current prime competitor) had just been released in March 2018.
Since that time, a few things have happened:
In June 2020, Enzyme switched from being maintained by Airbnb to being maintained by an individual.
In October 2020, React 17 was released.
Also in October 2020, an unofficial Enzyme adapter for React 17 was released.
In December 2021, the author of the unofficial adapter for React 17 wrote an article entitled Enzyme is Dead, where he stated that he would not be writing an adapter for React 18.
In March 2022, React 18 was released.
In April 2022, the Enzyme maintainer has stated that he will work on a React 17 adapter before a React 18 adapter.
As of April 2022, the official adapter for React 17 has not been released.
Why I currently recommend React Testing Library
React Testing Library and Enzyme do the same job: rendering components so that they can be tested with Jest. I recommend React Testing Library over Enzyme for these reasons:
Unlike Enzyme, React Testing Library does not rely on React internals. This means React Testing Library does not have an adapter that needs to be updated with each release of React, and is far less likely to have issues with new React versions.
I find React Testing Library to be easier to use than Enzyme.
What all this means for this course
Now that React 18 has come out and there's no React 17 adapter in sight, I don't believe there's a future for Enzyme. I would not recommend this course for people who are looking to learn how to test new React applications. Instead I would recommend you find a course on React Testing Library.
That said, there are still around 2.4 million downloads of Enzyme a week (as of April 2022). Here are the circumstances under which I would recommend learning Enzyme:
You are working on a legacy project that already has a large suite of Enzyme tests, and the project never intends to upgrade to React 18.
You are working on a project with a large suite of existing Enzyme tests, and you wish to understand these tests before migrating to React Testing Library (RTL). However, Wojciech Maj, the author of the unoffiical Enzyme adapter for React 17, suggests:
While Migrate from Enzyme support article is available, I suggest you to just start fresh, forgetting that Enzyme has ever existed. RTL is by no means an Enzyme drop-in replacement, so having a completely fresh mindset will help you getting the most of it.
The Bottom Line
Enzyme was a great way to test React applications when this course came out; however, I can no longer recommend it. I encourage you to study React Testing Library instead.
Bạn sẽ học được gì
Write unit, integration and functional tests for React, Hooks, Contex and Redux using Jest and Enzyme.
Know the tradeoffs for different testing approaches and when to choose which approach.
Plan your React app more effectively via Test Driven Development.
Mock methods and modules to keep your tests isolated.
Yêu cầu
- Basic Familiarity with JavaScript and React
Nội dung khoá học
Viết Bình Luận
Khoá học liên quan
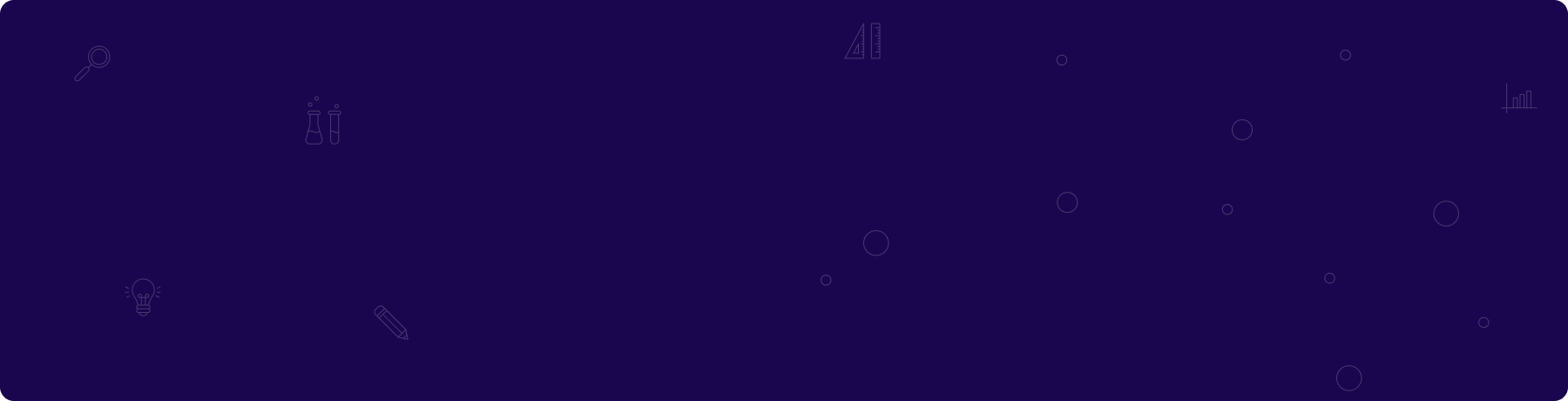
Đăng ký get khoá học Udemy - Unica - Gitiho giá chỉ 50k!
Get khoá học giá rẻ ngay trước khi bị fix.
Đánh giá của học viên
Bình luận khách hàng